本文最后更新于:2023年3月23日 上午
先是双色球规则
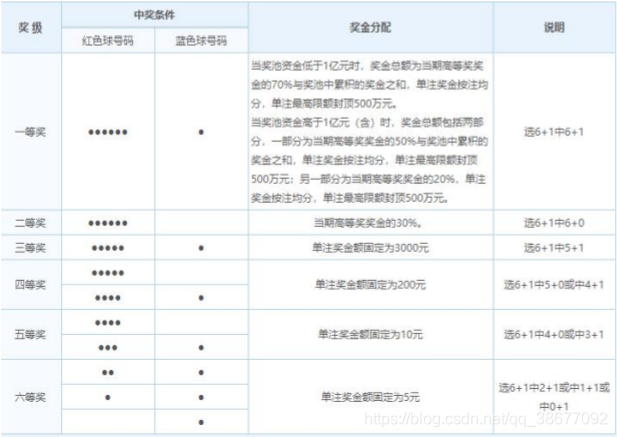
然后是老师当时讲的一个思路(虽然我自己也想到了)
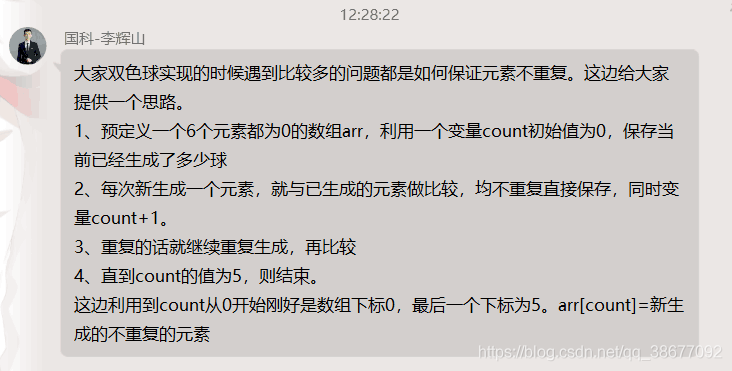
下面就是代码实现了,有C基础的同学应该很容易可以看懂,即使没有也没事,我自己脑子没那么好,写的就都是最简单脑子最能理解得来的方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307
| package cn.goktech;
import java.util.Random; import java.util.Scanner;
public class DoubleColorBall { public static void main(String[] args) { Random randomBlue = new Random(); int numBlue = randomBlue.nextInt(16)+1; System.out.println("numBlue="+numBlue); int[] ary=new int[6]; int count = 0; while(count<=5) { Random randomRed = new Random(); int numRed = randomRed.nextInt(33)+1; int flag = 0; for(int i=0;i<=5;i++) { if(numRed!=ary[i]) { flag++; } } if(flag==6) { ary[count]=numRed; count++; } } for(int j=0;j<=5;j++) { int a=ary[j]; System.out.println("ary["+j+"]="+a); } System.out.println("-----system information-----"); System.out.println(); System.out.println();
int scBlue; while(1==1) { Scanner sc1 = new Scanner(System.in); System.out.println("----请输入您的蓝球号码----"); System.out.print("请输入1~16的数字并按回车结束:"); scBlue = sc1.nextInt(); if(scBlue<1 || scBlue>16) { System.out.println("请输入1~16的数字!"); } else { System.out.println("用户输入的蓝球号码为:"+scBlue); break; } } int ccount = 0; int[] ary2=new int[6]; while(ccount<=5) { Scanner sc2 = new Scanner(System.in); int no=ccount+1; System.out.println("----请输入您的"+no+"号红球号码----"); System.out.print("请输入1~33的数字并按回车结束:"); int scRed = sc2.nextInt(); if(scRed<1 || scRed>33) { System.out.println("请输入1~33的数字!"); } else { System.out.println("用户输入的"+no+"号红球号码为:"+scRed); int flag2 = 0; for(int i=0;i<=5;i++) { if(scRed!=ary2[i]) { flag2++; } else { System.out.println("您输入了重复的号码!请重新输入!"); } } if(flag2==6) { ary2[ccount]=scRed; ccount++; } } } for(int k=0;k<=5;k++) { int a=ary2[k]; System.out.println("ary2["+k+"]="+a); } int Blue = 0; if(scBlue==numBlue) { Blue++; } int x; int Red=0; for(int l =0;l<=5;l++) { for(int m=0;m<=5;m++) { x=ary2[l]; if(x==ary[m]) { Red++; } } } double bonusAll=0; while(1==1) { Scanner sc3 = new Scanner(System.in); System.out.println("----请输入奖金池累计奖金----"); System.out.print("请输入大于等于0的数字并按回车结束:"); bonusAll = sc3.nextInt(); if(bonusAll<0) { System.out.println("请输入大于等于0的数字!你在搞笑吧!"); } else { System.out.println("奖金池累计奖金为:"+bonusAll); break; } } double bonusNow=0; while(1==1) { Scanner sc4 = new Scanner(System.in); System.out.println("----请输入本期高等奖奖金----"); System.out.print("请输入大于0的数字并按回车结束:"); bonusNow = sc4.nextInt(); if(bonusNow<=0) { System.out.println("请输入大于0的数字!你在搞笑吧!"); } else if(bonusNow>0 && bonusNow<=10) { System.out.println("奖金池累计奖金为:"+bonusNow); System.out.println("不是吧阿sir,我找辉山鸽鸽送我的都比你多,这点钱不配"); bonusNow=0; System.out.println("已清空奖金池累计奖金"); System.out.println("请重新输入"); } else if(bonusNow>10 && bonusNow<=10000) { System.out.println("奖金池累计奖金为:"+bonusNow); System.out.println("就这就这?就这?就这?你也太抠门了吧( ̄_, ̄ )"); break; } else if(bonusNow>10000 && bonusNow<=1000000) { System.out.println("奖金池累计奖金为:"+bonusNow); System.out.println("老板,给的太少了吧,下次给多点( ̄_, ̄ )"); break; } else { System.out.println("奖金池累计奖金为:"+bonusNow); break; } } System.out.println("您的奖赏等级为:"); System.out.println("这么激动人心的时刻就要到了!"); System.out.println("到底是多少呢?"); System.out.println("你猜猜看?"); System.out.println("你猜猜看?"); System.out.println("你猜猜看?");
System.out.println("别猜了!"); System.out.println("蓝球号码是:"+numBlue); for(int k=0;k<=5;k++) { int a=ary[k]; System.out.println("红球"+k+1+"号为:"+a); } System.out.println("蓝球中奖个数为:"+Blue); System.out.println("红球中奖个数为:"+Red);
System.out.println("您的奖赏等级为......"); if(Blue==1 && Red==6) { System.out.println("============"); System.out.println("=一等奖!!!!!!!!="); System.out.println("============"); int sameNote; while(1==1) {
Scanner sc5 = new Scanner(System.in); System.out.println("----请输入本期同注人数----"); System.out.print("请输入大于0的数字并按回车结束:"); sameNote = sc5.nextInt(); if(sameNote<0) { System.out.println("请输入大于等于0的数字!你在搞笑吧!"); } else { System.out.println("本期同注人数为:"+sameNote); break; } } double price1; if(bonusAll<100000000) { price1=(bonusNow*0.7+bonusAll)/sameNote; } else { price1=((bonusNow*0.5+bonusAll)+bonusNow*0.5)/sameNote; } if(price1>5000000) { price1=5000000; } System.out.println("你的奖金为:"+price1+"RMB"); System.out.println("恭喜恭喜!快!跟我五五开!"); double tmp1=price1*0.5; System.out.println("你"+tmp1+"RMB,我"+tmp1+"RMB"); } else if(Blue==0 && Red==6) { double price2; price2=bonusNow*0.3; System.out.println("二等奖!!"); System.out.println("你的奖金为:"+price2+"RMB"); System.out.println("恭喜恭喜!快!跟我四六开!"); double tmp2=price2*0.4,tmp3=price2*0.6; System.out.println("你"+tmp2+"RMB,我"+tmp3+"RMB"); } else if(Blue==1 && Red==5) { System.out.println("三等奖!!"); System.out.println("你的奖金为:3000块钱!"); System.out.println("好家伙!快!跟我五五开!你1k5我1k5!"); } else if((Blue==0 && Red==5)||(Blue==1 && Red==4)) { System.out.println("四等奖!!"); System.out.println("你的奖金为:200块钱!"); System.out.println("快!跟我三七开!200块钱你60我140!"); } else if((Blue==0 && Red==4)||(Blue==1 && Red==3)) { System.out.println("五等奖!!"); System.out.println("你的奖金为:10块钱!"); System.out.println("十块钱也是钱!快!白送我!"); } else if((Blue==1 && Red==2)||(Blue==1 && Red==1)||(Blue==1 && Red==0)) { System.out.println("六等奖!!"); System.out.println("你的奖金为:5块钱!"); System.out.println("5块钱!啊......才5块钱啊......"); System.out.println("算了,这次就送给你吧。下次中奖记得喊我啊。"); } else { System.out.println("鸭蛋奖!"); System.out.println("就你这运气还想脱非入欧?"); System.out.println("做梦吧!"); System.out.println("还搁这买彩票呐?"); System.out.println("先奖励自己拿一颗鸭蛋砸自己头上清醒一下吧!"); System.out.println("那"); System.out.println("掰掰"); } } }
|