本文最后更新于 2024年11月7日 下午
之前的SpringBoot期末复习中多包含了Redis和SpringCloud的知识点,现在划分开来方便复习
毕设就是用Spring Cloud整的,多看看还是比较好,虽然我已经写完了哈哈哈哈
SpringCloud(1-3简答、4-5选择、6编程)
1、微服务架构演进,课上前后讲了两遍,印象不深的去看视频(简答题重点考区)
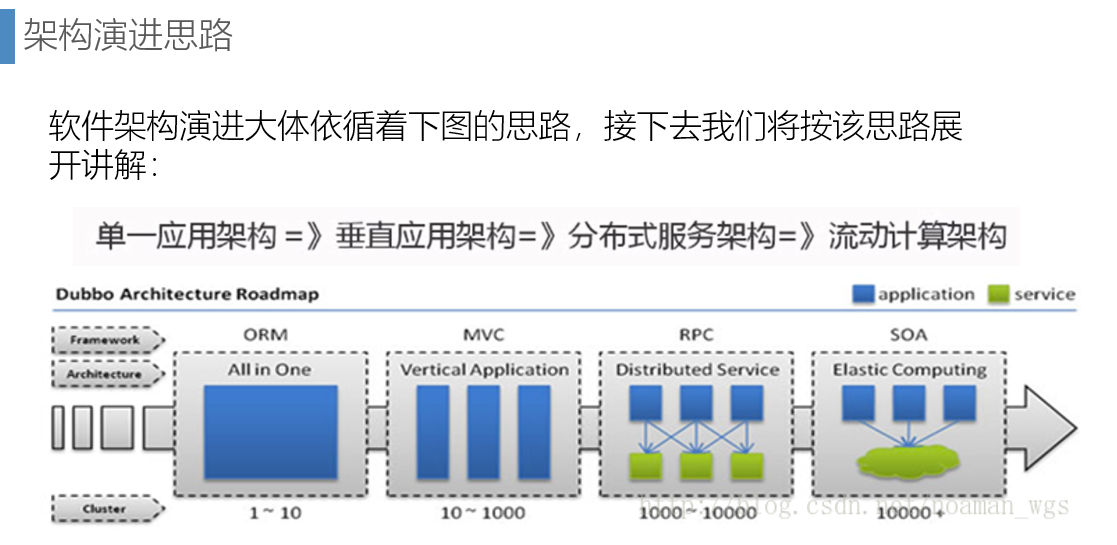
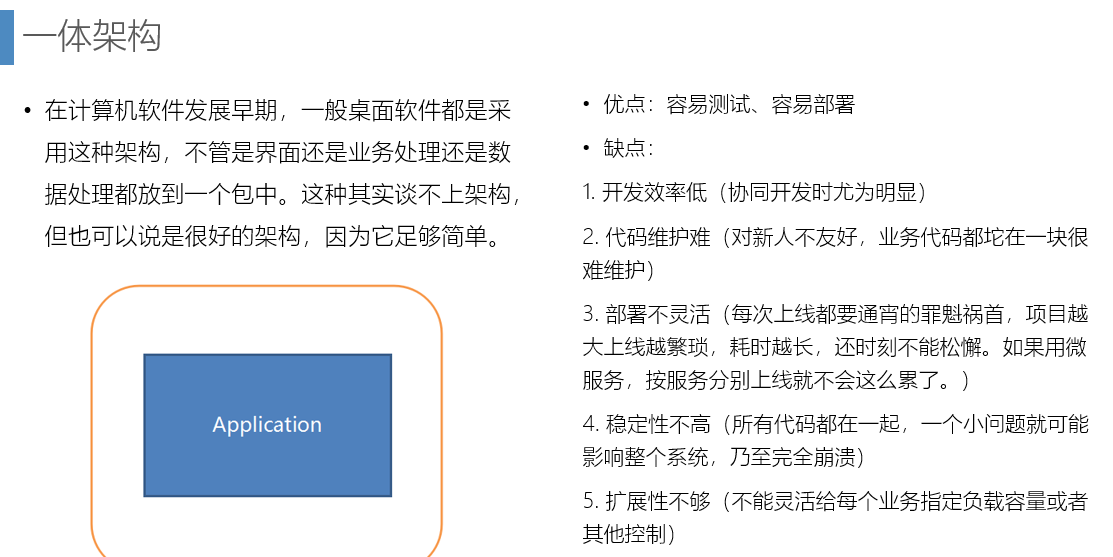
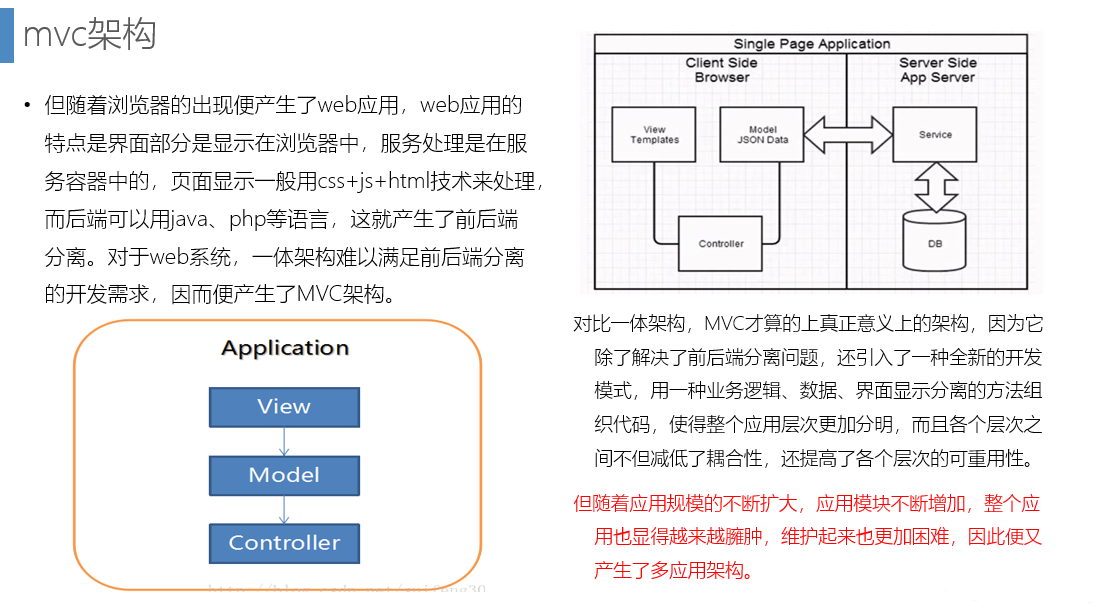
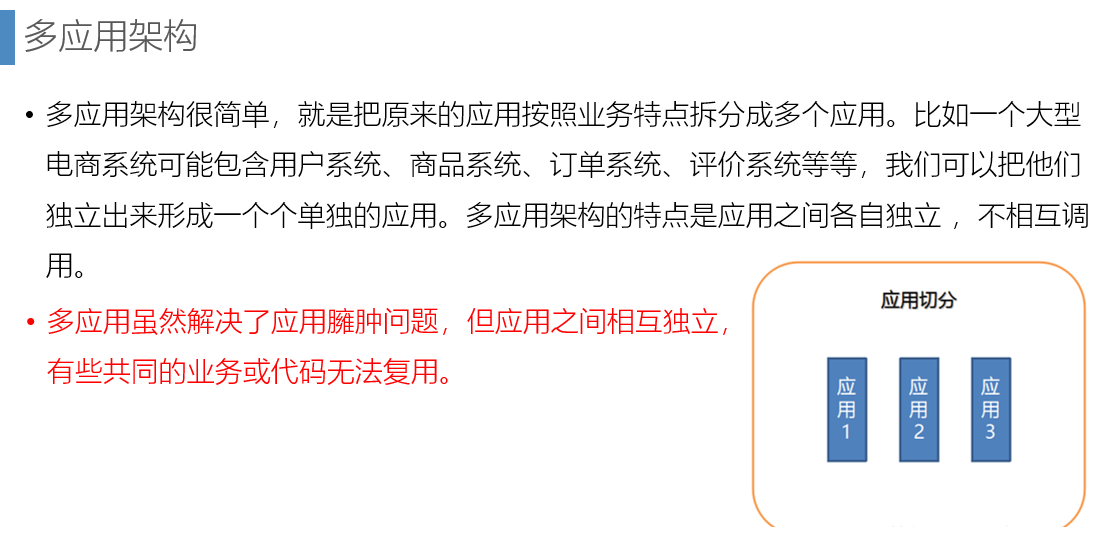
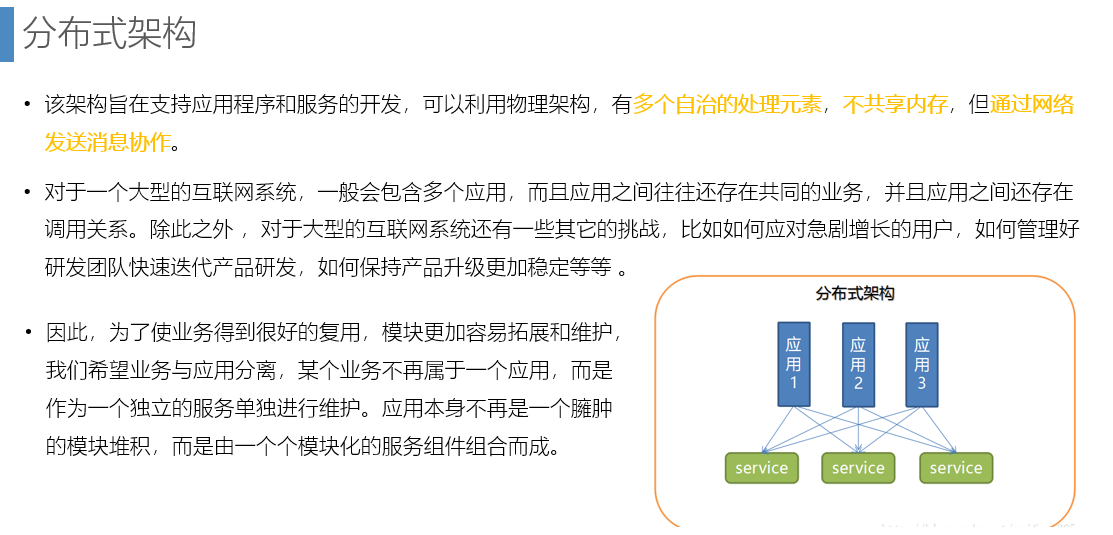
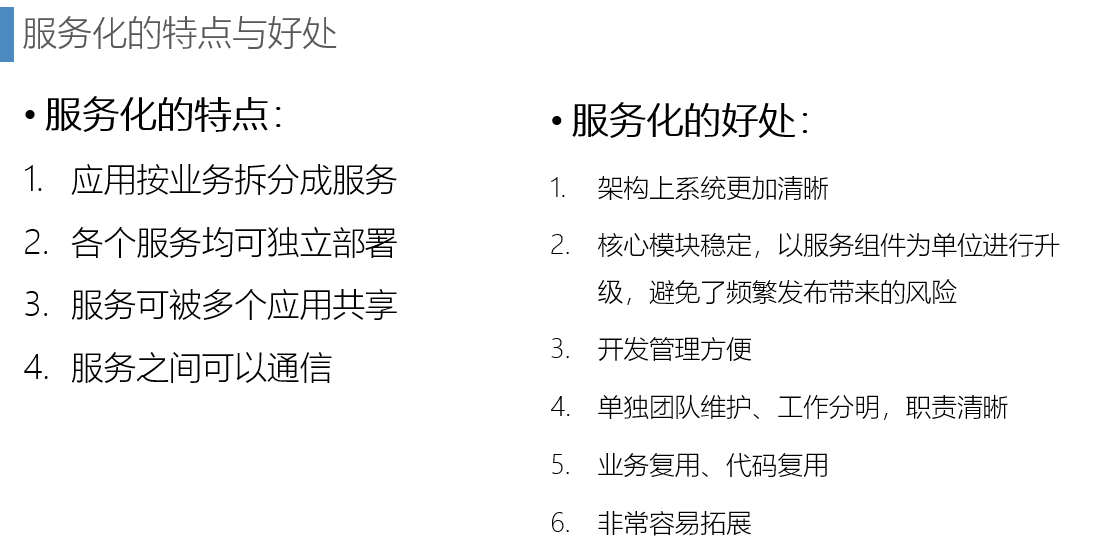
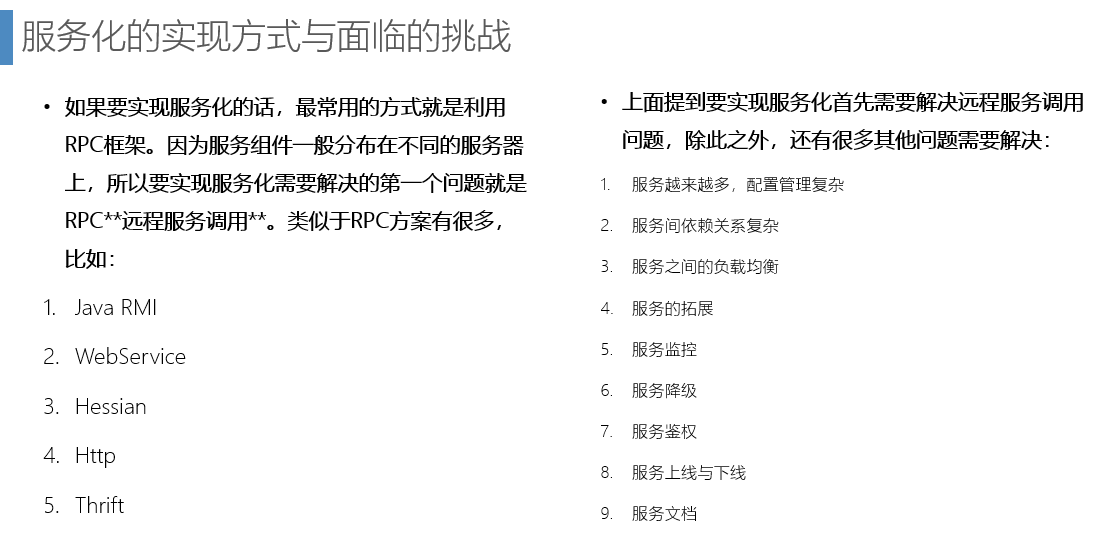
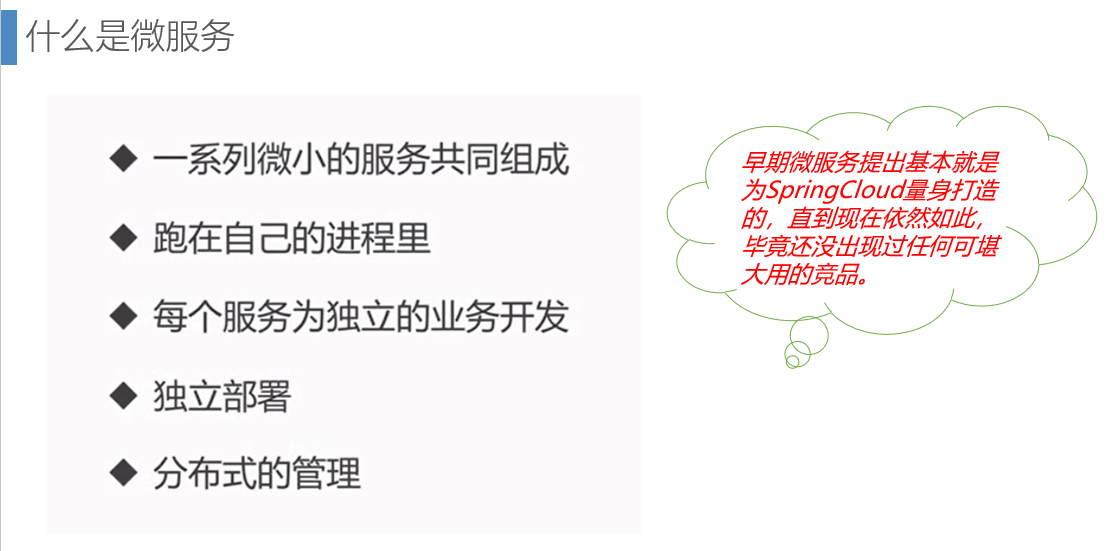
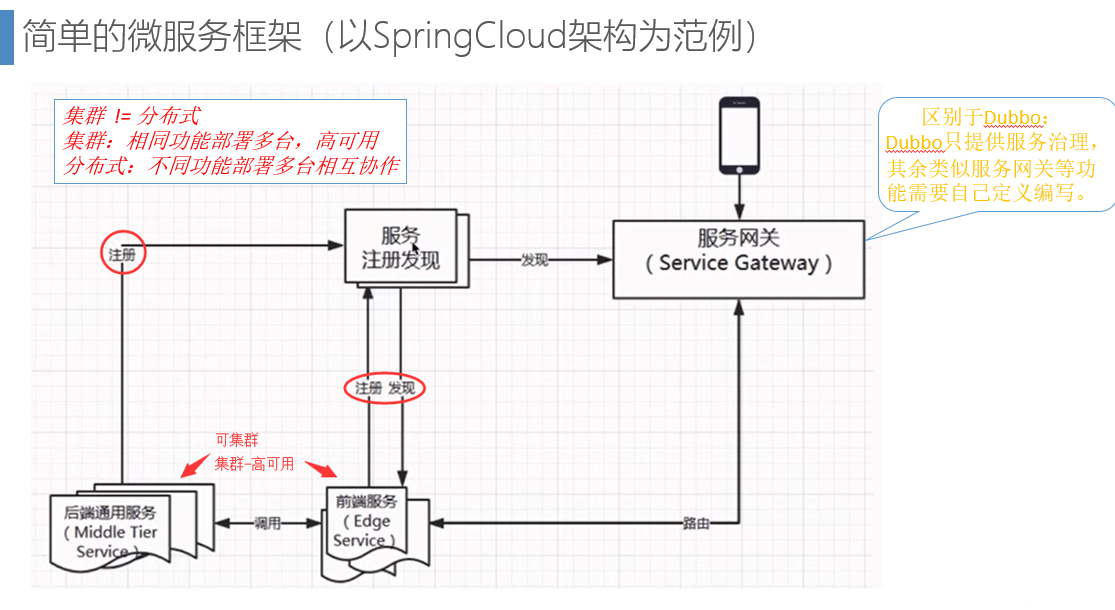
2、是什么,特点,优势
(1)是什么
Spring cloud是一个开发工具集,含多个子项目
- 利用spring Boot的开发便利
- 主要是基于对netfilix开源组件进行封装
Spring cloud 简化了分布式开发
(2)特点
Spring Cloud 是一套完整的微服务解决方案,基于 Spring Boot 框架,准确的说,它不是一个框架,而是一个大的容器,它将市面上较好的微服务框架集成进来,从而简化了开发者的代码量
(3)优势
- 集大成者
• Spring Cloud 包含了微服务架构的方方面面
- 约定优于配置
• 基于注解,没有配置文件
- 轻量级组件
• Spring Cloud 整合的组件大多比较轻量级,且都是各自领域的佼佼者
- 开发简便
• Spring Cloud 对各个组件进行了大量的封装,从而简化了开发
- 开发灵活
• Spring Cloud 的组件都是解耦的,开发人员可以灵活按需选择组件
3、知识沉淀——微服务学习过程中必须了解的5个问题
问题1:分布式系统中为什么需要注册中心?
问题2:服务多的时候,如何找到最适合我的服务?
问题3:客户端和服务端所用语言不同怎么办?
问题4:原有项目如何拆分成微服务项目?
问题5:哪些项目不适合演进成微服务架构?
问题1:分布式系统中为什么需要注册中心?
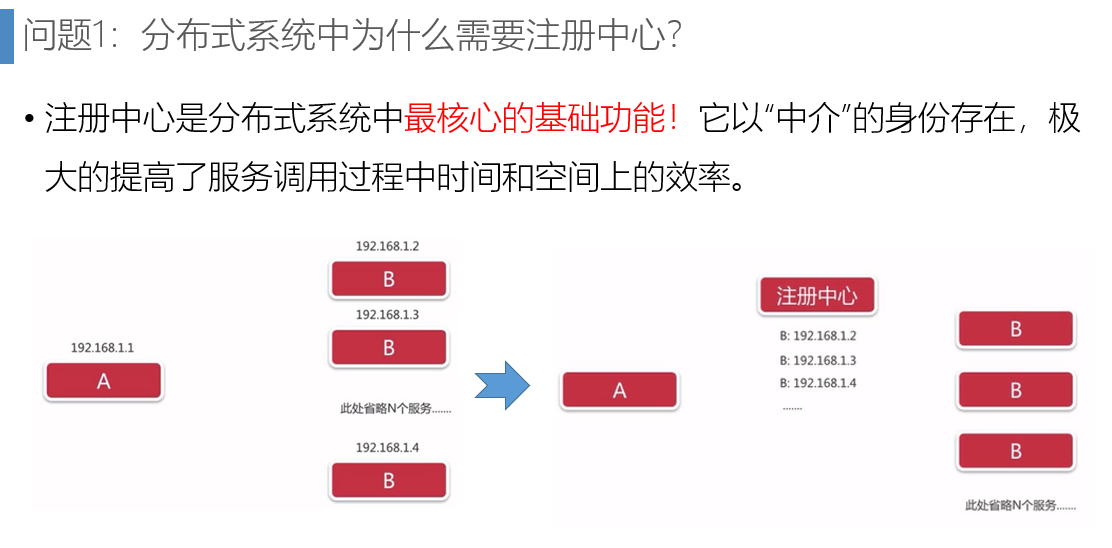
问题2:服务多的时候,如何找到最适合我的服务?
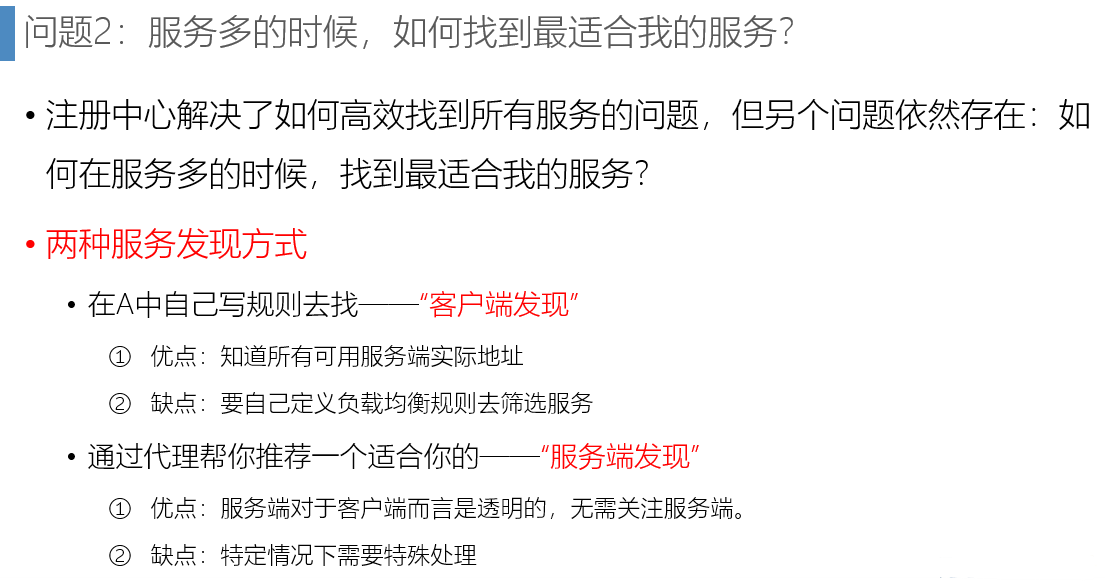
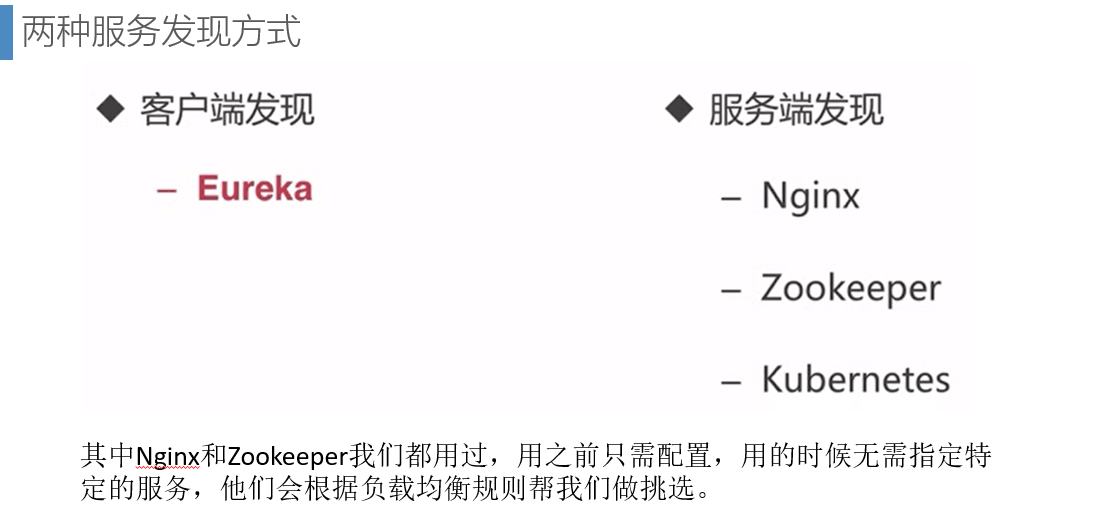
问题3:客户端和服务端所用语言不同怎么办?
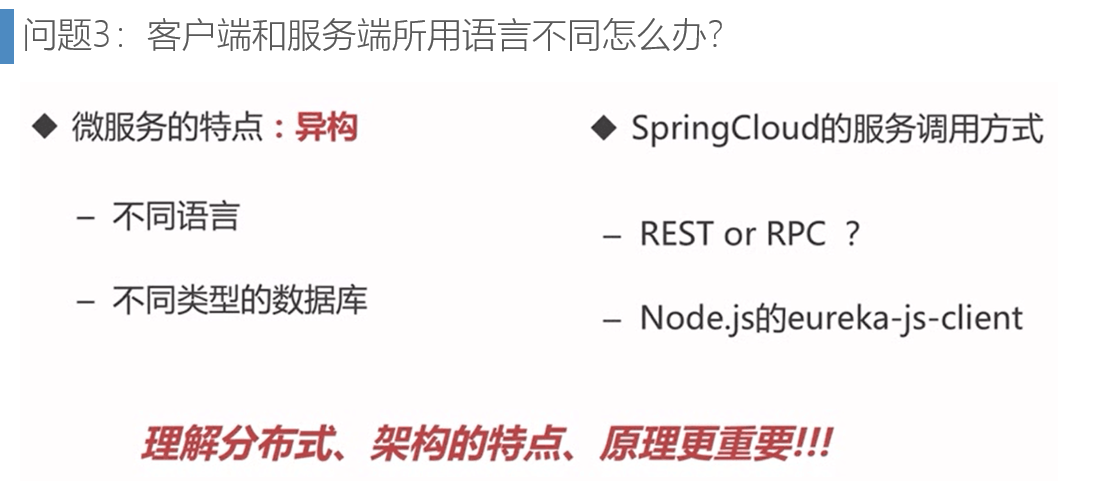
问题4:原有项目如何拆分成微服务项目?
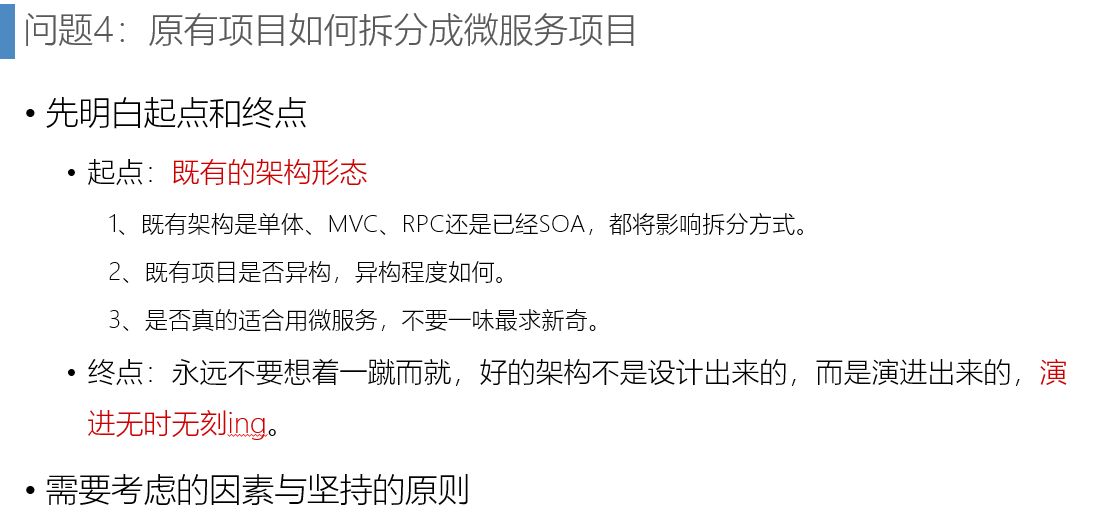
问题5:哪些项目不适合演进成微服务架构?
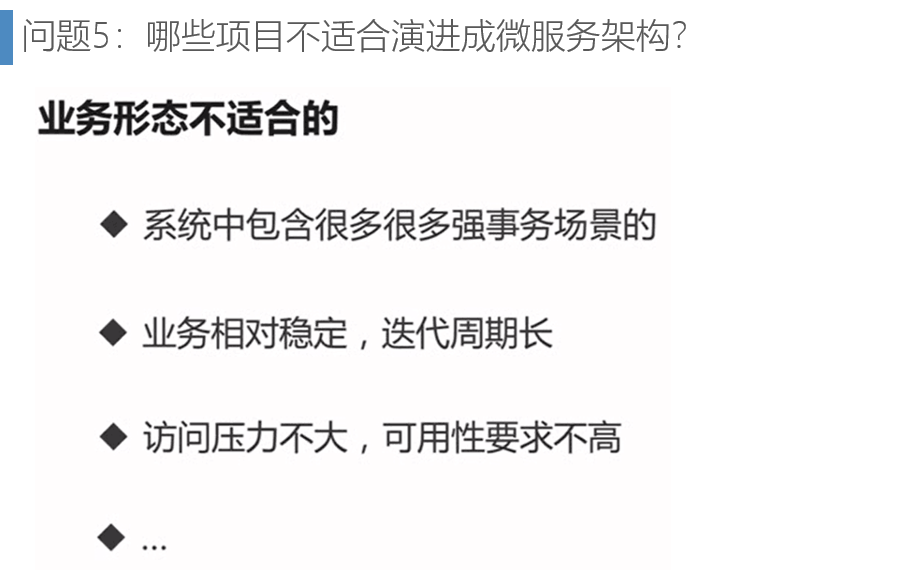
4、注册中心Eureka 配置、注解、运行效果、高可用!!
application.yml
1 2 3 4
| eureka: client: registerWithEureka: false fetchRegistry: false
|
application-e1.yml
1 2 3 4 5 6 7 8 9
| server: port: 8761 eureka: instance: hostname: eureka-1 client: service-url: defaultZone: http://eureka-2:8762/eureka/
|
application-e2.yml
1 2 3 4 5 6 7 8 9
| server: port: 8762 eureka: instance: hostname: eureka-2 client: service-url: defaultZone: http://eureka-1:8761/eureka/
|
5、服务消费者RestTemplate和Feign 配置、注解、运行效果
(1)RestTemplate
AppClient
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49
| package com.xxx.controller; @RestController public class ClientController { @Autowired private RestTemplate restTemplate;
@RequestMapping("getmsg") public String getMsg(String name) { String response = restTemplate.getForObject("http://APPSERVICE/hello?name="+name, String.class); return response; } @PostMapping("goods") public String addGood(@Valid Goods goods) throws Exception{
String response = restTemplate.postForObject("http://APPSERVICE/goods",goods, String.class); return response; } @PutMapping("goods") public void editGoods(Goods goods) {
restTemplate.put("http://APPSERVICE/goods",goods); } @DeleteMapping("goods/{gid}") public void removeGoods(@PathVariable int gid) { restTemplate.delete("http://APPSERVICE/goods/"+gid, String.class); } @GetMapping("goods/{gid}") public Goods findGood(@PathVariable int gid) { Goods response = restTemplate.getForObject("http://APPSERVICE/goods/"+gid, Goods.class); return response; }
}
|
AppService
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56
| @RestController public class GoodsController { @Value("${server.port}") private int port; @Autowired private GoodsService goodsService; @PostMapping("goods") public String addGoods(@RequestBody Goods goods,BindingResult br) { if(br.hasErrors()) { return br.getFieldError().getDefaultMessage(); }else { System.out.println(goods.getGname()); System.out.println(goods.getGname()); System.out.println(goods.getGname()); System.out.println(goods.getGid()); goodsService.addGoods(goods); return "添加成功"; } } @PutMapping("goods") public String editGoods(@RequestBody Goods goods) { goodsService.editGoods(goods); return "修改成功"; } @DeleteMapping("goods/{gid}") public String removeGoods(@PathVariable int gid) { goodsService.removeGoods(gid); return "删除成功"; } @GetMapping("goods/less/{price}/{type}") public List<Goods> findGoods(@PathVariable double price,@PathVariable String type){ GoodsExample ge = new GoodsExample(); ge.createCriteria().andTypeEqualTo(type).andPriceLessThan(price); return goodsService.findByCondition(ge); } @GetMapping("goods/{gid}") public Goods findGood(@PathVariable int gid){ return goodsService.findByGid(gid); }
}
|
AppClientApplication
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| package com.xxx;
@EnableEurekaClient @SpringBootApplication public class AppClientApplication {
public static void main(String[] args) { SpringApplication.run(AppClientApplication.class, args); }
@Bean @LoadBalanced public RestTemplate getRestTemplate() { RestTemplate restTemplate = new RestTemplate(); return restTemplate; } }
|
(2)Feign
1 2 3 4 5 6 7 8 9 10 11 12
| package com.xxx; @EnableEurekaClient @EnableFeignClients @SpringBootApplication public class FeignClientApplication {
public static void main(String[] args) { SpringApplication.run(FeignClientApplication.class, args); }
}
|
1 2 3 4 5 6 7 8
| package com.xxx.client; @FeignClient("APPSERVICE") public interface HelloClient {
@RequestMapping("hello") public String sayHello(@RequestParam("name") String name); }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| package com.xxx.controller; @RestController public class ClientController { @Autowired private HelloClient helloClient; @RequestMapping("msg") public String getMsg(String name) { String response = helloClient.sayHello(name); return response; } @RequestMapping("/hi") public String sayHi() { return "hi"; } }
|
6、SpringCloud编程题只靠配置文件写法,不考Java代码
(1)AppClient
application.yml
1 2 3 4 5 6 7 8 9 10 11
| server: port: 8888 spring: application: name: appclient eureka: client: service-url: defaultZone: http://eureka-1:8761/eureka/
|
(2)AppService
application.yml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| eureka: client: service-url: defaultZone: http://eureka-1:8761/eureka/
spring: application: name: appservice datasource: url: jdbc:mysql://localhost:3306/shop?characterEncoding=utf8&useSSL=false&serverTimezone=Asia/Shanghai&rewriteBatchedStatements=true username: root password: 123456 driver-class-name: com.mysql.cj.jdbc.Driver mybatis: mapper-locations: classpath:com/etc/mapper/xml/GoodsMapper.xml
|
application-as1.yml
application-as2.yml
application-as3.yml
(3)EurekaServer-ha
application.yml
1 2 3 4
| eureka: client: registerWithEureka: false fetchRegistry: false
|
application-e1.yml
1 2 3 4 5 6 7 8 9
| server: port: 8761 eureka: instance: hostname: eureka-1 client: service-url: defaultZone: http://eureka-2:8762/eureka/
|
application-e2.yml
1 2 3 4 5 6 7 8 9
| server: port: 8762 eureka: instance: hostname: eureka-2 client: service-url: defaultZone: http://eureka-1:8761/eureka/
|
题型:
单选:10-15题 (每题2分)
多选:5-8题(每题3分)
判断:0-10题(每题1分)
简答:6-8题(每题6分)
编程:1-2题(每题10分)